Day 2 AI bootcamp: Building a News App Using SearchAPI
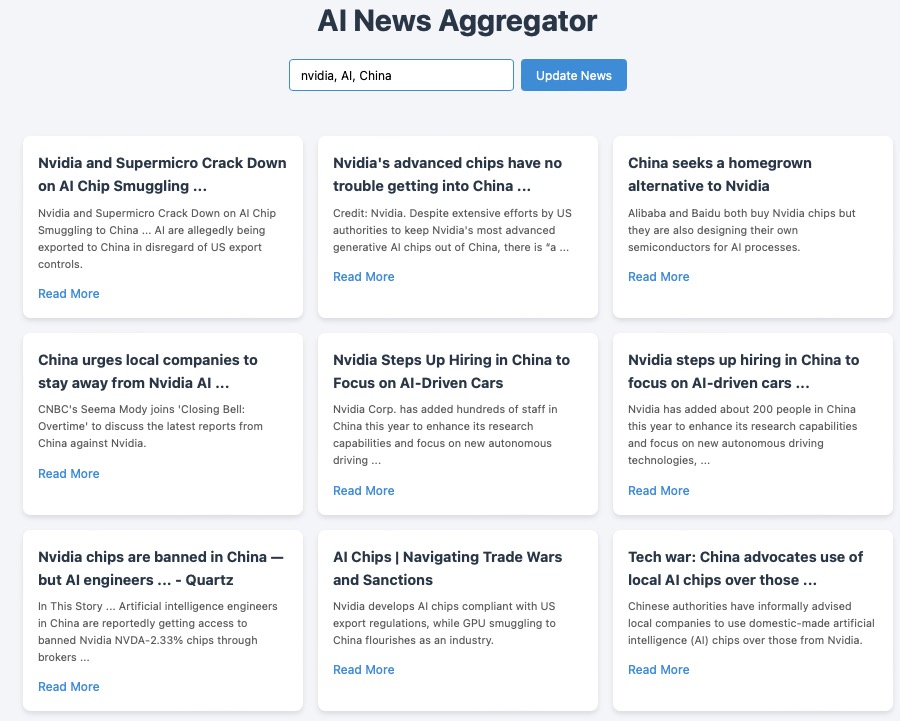
Developing an application that collects and curates news articles tailored to individual user interests involves a blend of advanced technologies, including web development, data processing, and artificial intelligence. This guide provides a detailed approach to creating a personalized news aggregator, emphasizing the use of SearchAPI for fetching news articles. By integrating AI tools and considering optimal hosting solutions, you can build a robust application that delivers relevant content to users.
Conceptual Overview
Creating a personalized news aggregator requires careful planning and the integration of multiple components:
- Data Collection: Gathering news articles using SearchAPI.
- User Profile Management: Storing and analyzing user preferences and interaction history.
- Recommendation Engine: Employing AI algorithms to match news content with user interests.
- User Interface: Designing an intuitive interface for user interaction.
- Deployment and Hosting: Selecting reliable hosting services to ensure optimal performance.
Setting Up the Development Environment
Begin by preparing your development environment with the necessary tools and technologies.
- Programming Language: Python is ideal due to its extensive libraries and strong community support.
- Web Framework: Use Flask or Django for backend development.
- Database Management: Implement PostgreSQL or MongoDB to store user data and articles.
- AI and Machine Learning Libraries: Utilize TensorFlow or PyTorch for developing recommendation algorithms.
- Front-End Technologies: Employ HTML, CSS, and JavaScript frameworks like React.js or Vue.js for the user interface.
Implementing the Application
-
Initializing the Flask Application
Start by setting up a basic Flask application to handle web requests.
from flask import Flask, render_template, request, jsonify app = Flask(__name__) @app.route('/') def home(): return render_template('home.html') if __name__ == '__main__': app.run(debug=True)
-
Fetching News Articles Using SearchAPI
Instead of using NewsAPI, integrate SearchAPI.io to retrieve news articles. Ensure you have an API key from SearchAPI.io.
import requests def fetch_news(keywords): api_key = 'YOUR_SEARCHAPI_KEY' endpoint = 'https://api.searchapi.io/api/v1/news/search' headers = { 'x-api-key': api_key } params = { 'q': keywords, 'language': 'en', 'page': 1, 'pageSize': 20 } response = requests.get(endpoint, headers=headers, params=params) if response.status_code == 200: data = response.json() articles = data.get('articles', []) return articles else: return []
This function fetches news articles based on the provided keywords using the correct SearchAPI.io endpoint and authentication header.
-
Designing the Database Schema
Create a database to store user profiles and articles.
-
User Table:
-
user_id: Unique identifier for each user.
-
preferences: List of user interests (e.g., technology, sports).
-
interaction_history: Records of user interactions with articles (e.g., likes, shares).
-
-
Article Table:
-
article_id: Unique identifier for each article.
-
title: Title of the article.
-
content: Main text content.
-
url: Link to the original article.
-
published_at: Timestamp of publication.
-
source: Source of the article.
-
-
-
Building the Recommendation Engine
Implement a content-based filtering system using machine learning to recommend articles that match user preferences.
from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.metrics.pairwise import linear_kernel import pandas as pd def create_user_profile(user_preferences): return ' '.join(user_preferences) def recommend_articles(user_profile, articles): df = pd.DataFrame(articles) tfidf = TfidfVectorizer(stop_words='english') tfidf_matrix = tfidf.fit_transform(df['content']) user_tfidf = tfidf.transform([user_profile]) cosine_similarities = linear_kernel(user_tfidf, tfidf_matrix).flatten() related_docs_indices = cosine_similarities.argsort()[:-6:-1] return df.iloc[related_docs_indices]
This code snippet creates a user profile based on their preferences and computes the cosine similarity between the user profile and articles to recommend the most relevant ones.
-
Developing the User Interface
Design a responsive front-end interface that displays recommended articles and allows user interaction.
<!-- home.html --> <!DOCTYPE html> <html> <head> <title>Personalized News Aggregator</title> <link rel="stylesheet" href="static/styles.css"> </head> <body> <div id="app"> <h1>Welcome to Your Personalized News Feed</h1> <div id="articles"></div> </div> <script src="static/app.js"></script> </body> </html>
In your app.js, fetch recommended articles from the backend and display them:
fetch('/api/recommendations') .then(response => response.json()) .then(data => { const articlesDiv = document.getElementById('articles'); data.forEach(article => { const articleElem = document.createElement('div'); articleElem.className = 'article'; const title = document.createElement('h2'); title.textContent = article.title; const content = document.createElement('p'); content.textContent = article.content.substring(0, 200) + '...'; const link = document.createElement('a'); link.href = article.url; link.textContent = 'Read more'; link.target = '_blank'; articleElem.appendChild(title); articleElem.appendChild(content); articleElem.appendChild(link); articlesDiv.appendChild(articleElem); }); });
-
Integrating AI Tools
Utilize AI tools to enhance content recommendations and personalize the user experience.
- Natural Language Processing (NLP): Leverage NLP techniques to better understand article content and user interests.
- Machine Learning Models: Implement models that learn from user interactions to improve future recommendations.
Example: Using a Machine Learning Model for User Preferences
from sklearn.neighbors import NearestNeighbors def train_model(interaction_data): model = NearestNeighbors(metric='cosine', algorithm='brute') model.fit(interaction_data) return model def get_similar_users(model, user_vector): distances, indices = model.kneighbors([user_vector], n_neighbors=5) return indices.flatten()
-
Deploying the Application
Choose a hosting service that meets the scalability and performance needs of your application.
- InterServer: Offers reliable VPS and dedicated server solutions with instant setup and competitive pricing. Check InterServer plans here
- Contabo: Provides high-performance VPS with generous resources at affordable prices. Explore Contabo VPS options
- Web Hosting Pad: Suitable for web applications requiring cost-effective hosting solutions. View Web Hosting Pad services
Affiliate Disclosure: By using the links above, you support our project at no additional cost to you.
-
Enhancing Security and Privacy
Protect user data and ensure secure transactions.
- SSL Encryption: Secure data transmission with SSL certificates.
- Secure Coding Practices: Sanitize inputs and use parameterized queries to prevent SQL injection.
- Security Tools: Integrate services like Sucuri to safeguard your application against threats. Learn more about Sucuri
Additionally, consider offering VPN services to enhance user privacy when accessing news content.
- HideMy.Name VPN: Provides secure and anonymous browsing. Get HideMy.Name VPN here
-
Optimizing for Search Engines
Improve your application's visibility and accessibility.
- SEO Best Practices: Use meta tags, descriptive URLs, and semantic HTML.
- Performance Optimization: Minimize load times by optimizing images and code.
- Mobile Responsiveness: Ensure the application functions well on all devices.
Flowchart of Application Workflow
- User Authentication: The user logs in or registers, providing preferences.
- Data Retrieval: The application fetches news articles using SearchAPI based on user interests.
- Data Processing: Articles are analyzed and ranked using AI algorithms.
- Content Delivery: Recommended articles are displayed to the user.
- User Interaction: The user reads, likes, or saves articles.
- Feedback Loop: User interactions are recorded, updating their profile and improving future recommendations.
- Continuous Learning: The system refines its algorithms based on accumulated data.
Key Insights
The development of a personalized news aggregator leverages advanced technologies to deliver content that resonates with users. By integrating SearchAPI for data sourcing and applying AI for personalization, developers can create a dynamic and engaging application. Ensuring security, optimizing for SEO, and choosing the right hosting services are crucial steps in building a successful platform.
References
- Aggarwal, C. C. (2016). Recommender Systems: The Textbook. Springer.
- Jurafsky, D., & Martin, J. H. (2020). Speech and Language Processing (3rd ed.). Draft.
- Chollet, F. (2018). Deep Learning with Python. Manning Publications.
Further Learning Resources
For those interested in delving deeper into AI and machine learning applications in web development, consider exploring these books:
- "Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow" by Aurélien Géron: A practical guide to machine learning in Python. Buy on Amazon
- "Flask Web Development" by Miguel Grinberg: Learn how to build web applications in Python using Flask. Buy on Amazon
Affiliate Disclosure: Purchases made through these links may earn us a commission.
Conclusion
Building an AI-powered personalized news aggregator is a multifaceted endeavor that combines data science, machine learning, and web development. By utilizing SearchAPI for reliable news data and integrating AI tools for personalization, you can create a platform that continuously adapts to user interests. This guide serves as a foundation for developing such an application, emphasizing the importance of user experience, security, and scalability.
By focusing on the technical aspects and integrating practical code examples, this guide aims to assist developers and students in understanding the process of building a personalized news aggregator. Incorporating affiliate links provides an opportunity to support the project while offering valuable resources to readers.
Comments
Please log in to leave a comment.
No comments yet.